Calling ASP MVC Controllers from jQuery Ajax
Using ASP MVC Controllers as a source for Ajax is one of the easiest methods around. I’ll give you four simple examples of calling a controller function, show you how to pass parameters both ways and then show how to call and display a Partial View.
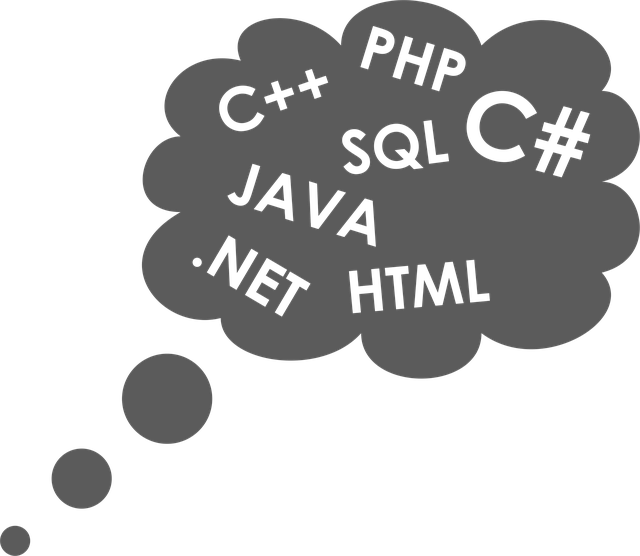
Using ASP MVC Controllers as a source for Ajax is one of the easiest methods around. I’ll give you four simple examples of calling a controller function, show you how to pass parameters both ways and then show how to call and display a Partial View. You can find the full source of my Visual Studio project here but if you have any MVC project available you can use it as long as you have jQuery available.
We’re going to test this by placing a <div> on the screen with a button, and then capture the button click to invoke the jQuery .ajax command to call the MVC Controller and display the result back in the div. So we basically have:
<div id="testarea"></div> <input id="btn1" type="button" value="Test 1" /> <input id="btn2" type="button" value="Test 2" /> <input id="btn3" type="button" value="Test 3" /> <input id="btn4" type="button" value="Test 4" />
In the Controller we can have the most basic function. The only thing extra here is that you must decorate the function with the directive [HttpPost] to tell our program to expect the call to be POSTed to us. If you don’t add this then it’s not going to work.
[HttpPost] public void Test01() { string notUsedString = "We got to Test01"; }
We’re not using the string, it’s just added so that you can put a breakpoint on it and confirm that the call is working.
Next we will switch to our output file, it can be a View or just a HTML page somewhere else. The jQuery will look like this:
$("#btn1").click(function () { $.ajax({ url: "/Home/Test01", datatype: "text", type: "POST", success: function (data) { $('#testarea').html("All OK"); }, error: function () { $("#testarea").html("ERROR"); } }); });
We capture the click event from btn1 and call the URL /Home/Test01. This is the Home Controller and function Test01. If your page is external to the project you should use a full URL address. In this case, .ajax function uses POST (although in this example we are not passing any parameters). In return we get 2 callback functions, success and error. In all cases we will just write anything we get back to the div with id of testarea.
Next, let’s do a similar call but let the controller function return some text.
[HttpPost] public string Test02() { // call and return a string return "Hello from Test02"; }
The jQuery code is only slightly different:
$("#btn2").click(function () { $.ajax({ url: "/Home/Test02", datatype: "text", type: "POST", success: function (data) { $('#testarea').html(data); }, error: function () { $("#testarea").html("ERROR"); } }); });
This time whatever data is returned by the success function will be written to the testarea div.
In Test 3 we’ll send some data to the controller and then get the same data back.
[HttpPost] public string Test03( string str1, string str2 ) { // call with two parameters and return them back return String.Format( "Test03: String1={0}; String2={1}", str1, str2 ); }
The names of the parameters used in the controller must be used by the Ajax call:
$("#btn3").click(function () { $.ajax({ url: "/Home/Test03", datatype: "text", data: { 'str1': 'qwerty', 'str2': 'its magic' }, type: "POST", success: function (data) { $('#testarea').html(data); }, error: function () { $("#testarea").html("ERROR"); } }); });
If you’re working at this stage then sky’s the limit as this covers most needs. The datatype can be set to json if your function is returning JSON, but instead of public string Test03 use public JsonResult Test03.
The last example is return a Partial View. Create a partial view named _MyPartialView and make it simple like:
<div style="height:100px;width:200px;background-color:#ccc;border:1px solid red;padding:10px;"> You have called a partial view. </div>
The Controller has a simple function that will be of type PartialViewResult.
[HttpPost] public PartialViewResult Test04() { // in practice you may need to set view parameters here return PartialView( "_MyPartialView" ); }
The jQuery .ajax call is as before
$("#btn4").click(function () { $.ajax({ url: "/Home/Test04", datatype: "text", type: "POST", success: function (data) { $('#testarea').html(data); }, error: function () { $("#testarea").html("ERROR"); } }); });
By combining all these techniques you should be able to easily create powerful Ajax-enabled websites.
Using unused variables as a location to place a breakpoint can be frustrating if the compiler optimises them away.
An alternative that I use is to output a trace message instead, this will never be optimised away.
Trace.WriteLine(“I’m alive!!”);
simple & brilliant, thank you